In this post, we will learn how to make a custom full screen dialog in android like the one showed below.
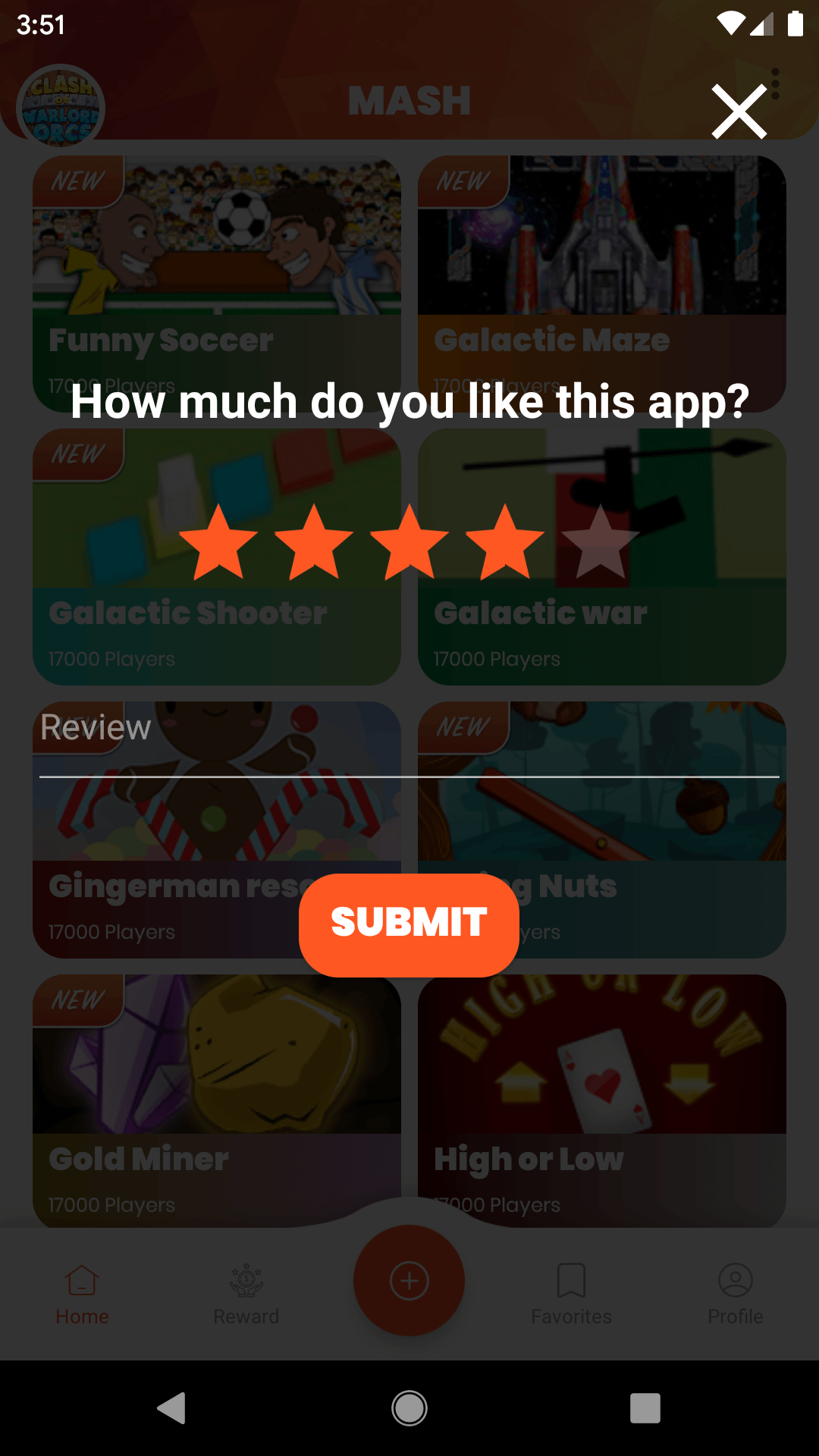
Creating full-screen dialog in android is a difficult task, even more, difficult if it has a custom layout. So, in this post, we will learn how to make a full-screen dialog in android, the easy way without even using any dialog!
First, create an empty activity. We called it RatingDialog
, you can call it whatever you want. Then open your styles.xml and add the below theme.
<style name="Theme.Transparent" parent="Theme.MaterialComponents.NoActionBar"> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> <item name="android:windowIsTranslucent">true</item> <item name="android:windowBackground">@android:color/transparent</item> <item name="android:windowContentOverlay">@null</item> <item name="windowNoTitle">true</item> <item name="android:windowIsFloating">false</item> <item name="android:backgroundDimEnabled">false</item> </style>
Next, open the manifests.xml and add the theme to your activity.
<activity android:name=".ui.dialogs.RatingDialog" android:theme="@style/Theme.Transparent"></activity>
Now, we will edit the layout of our theme.
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#CC000000" > <LinearLayout android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" > <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="How much do you like this app?" style="@style/TextAppearance.AppCompat.Headline" android:layout_gravity="center" android:textAlignment="center" android:paddingBottom="32dp" android:textStyle="bold" android:textColor="#FFFFFF" /> <RatingBar android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:paddingBottom="16dp" android:id="@+id/rating_ratingbar" /> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="32dp" android:layout_marginLeft="16dp" android:layout_marginRight="16dp" > <com.google.android.material.textfield.TextInputEditText android:layout_width="match_parent" android:layout_height="wrap_content" android:lines="2" android:textColor="#FFFFFF" android:id="@+id/rating_edit_text" android:hint="Review" /> </com.google.android.material.textfield.TextInputLayout> <androidx.cardview.widget.CardView android:id="@+id/collect_btn" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_margin="8dp" android:clickable="true" android:focusable="true" android:foreground="?attr/selectableItemBackground" app:cardBackgroundColor="@color/colorAccent" app:cardCornerRadius="20dp" app:cardElevation="2dp" android:layout_gravity="center" > <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:layout_marginLeft="16dp" android:layout_marginTop="8dp" android:layout_marginRight="16dp" android:layout_marginBottom="8dp" android:fontFamily="@font/poppins_black" android:text="Submit" android:textAlignment="center" android:textAllCaps="true" android:textColor="@android:color/white" android:textSize="20sp" /> </androidx.cardview.widget.CardView> </LinearLayout> <ImageView android:layout_width="48dp" android:layout_height="48dp" android:src="@drawable/ic_close_black_24dp" android:layout_gravity="right" android:layout_marginTop="32dp" android:layout_marginRight="16dp" android:tint="#FFFFFF" android:id="@+id/rating_close" /> </FrameLayout>
Note: We are using cardview to create the rounded buttons. You can use the regular button if you want.
Now, open the java file of our activity and add the following lines to make it full screen.
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) { getWindow().getDecorView().setSystemUiVisibility( View.SYSTEM_UI_FLAG_LAYOUT_STABLE | View.SYSTEM_UI_FLAG_LAYOUT_FULLSCREEN); }
That’s it, our full screen dialog is ready.
Let us know in comments if you are having any question regarding this Android Custom Full Screen Dialog.
And if you found this post helpful, then please help us by sharing this post with your friends learning Android Application Development. Thank You